Thanks for coming to the definitive resource for learning PHP, the flexible and potent programming language that powers a large portion of the modern web. Regardless of your level of expertise as a developer, this thorough course will take you from the basics to more complex ideas, giving you the knowledge and self-assurance you need to create dynamic and reliable online apps.
Gaining expertise in web building through step-by-step instruction in PHP is highly recommended. Here is a thorough lesson overview to help you navigate the procedure:
Step 1: Getting Started
Introduction to PHP
- Overview of PHP.
- History and purpose.
- Advantages and use cases.
Setting Up Your Environment
- Installing a local server (e.g., XAMPP, WAMP, or MAMP).
- Configuring PHP.
- Choosing a text editor or IDE (Integrated Development Environment).
Step 2: Basics of PHP
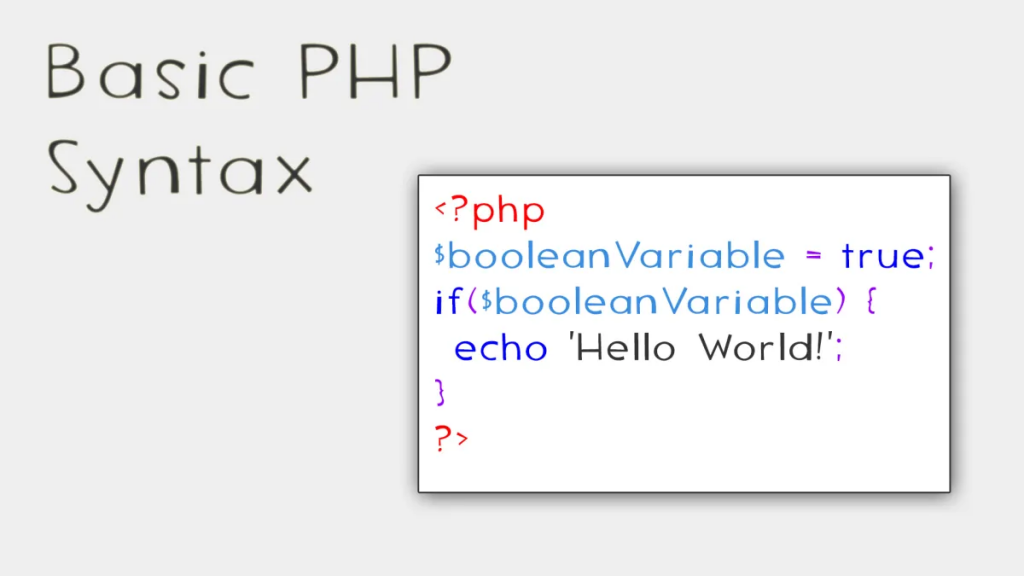
- Syntax and Structure
- Writing your first PHP script.
- Basic syntax rules.
- Comments in PHP.
- Variables and Data Types
- Declaring variables.
- Data types (string, integer, float, boolean, array, object).
- Variable scope.
- Operators
- Arithmetic operators.
- Comparison operators.
- Logical operators.
- Assignment operators.
Step 3: Control Structures
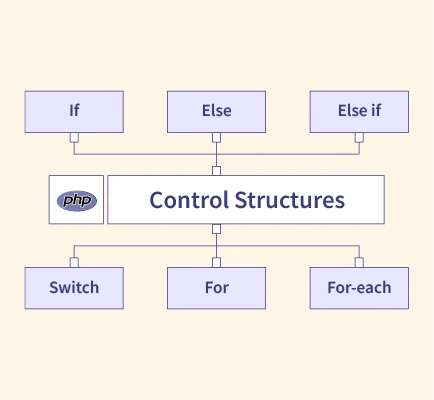
- Conditional Statements
- If statement.
- Else and elseif statements.
- Switch statement.
- Looping Structures
- While loop.
- For loop.
- Foreach loop.
- Loop control statements (break, continue).
Step 4: Functions and Arrays
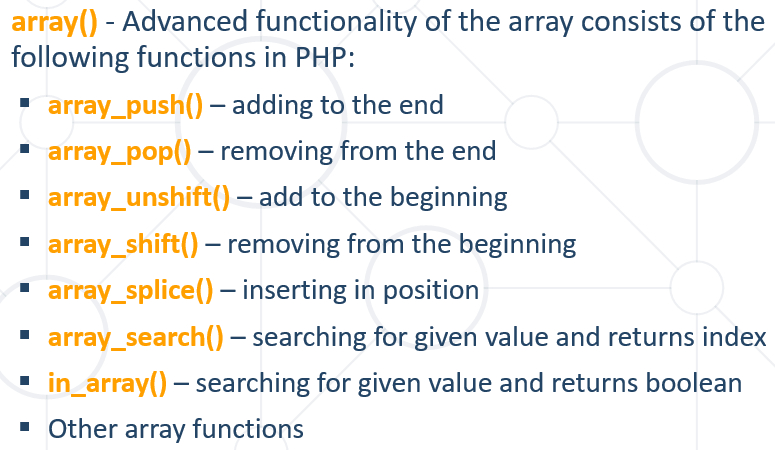
- Functions
- Declaring and calling functions.
- Function parameters and return values.
- Built-in functions vs. user-defined functions.
- Arrays
- Indexed arrays.
- Associative arrays.
- Multidimensional arrays.
- Array functions (e.g., array_push, array_pop, array_merge).
Step 5: Working with Forms
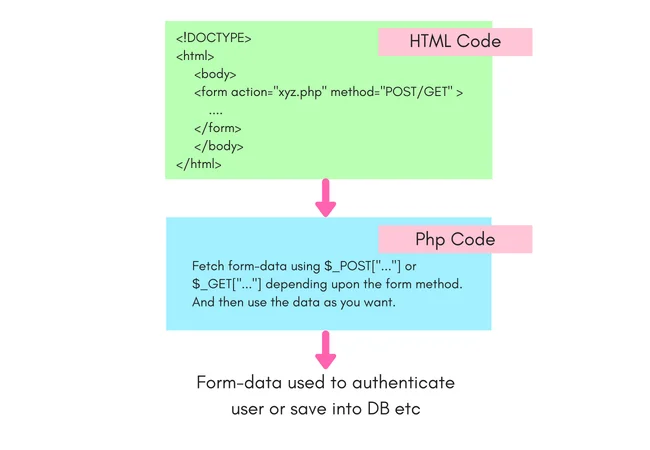
- Handling Form Data
- GET vs. POST methods.
- Accessing form data using $_GET and $_POST.
- Form validation and sanitization.
- Form Processing
- Building dynamic forms.
- Processing form data.
- Form submission best practices.
Step 6: Working with Files and Databases
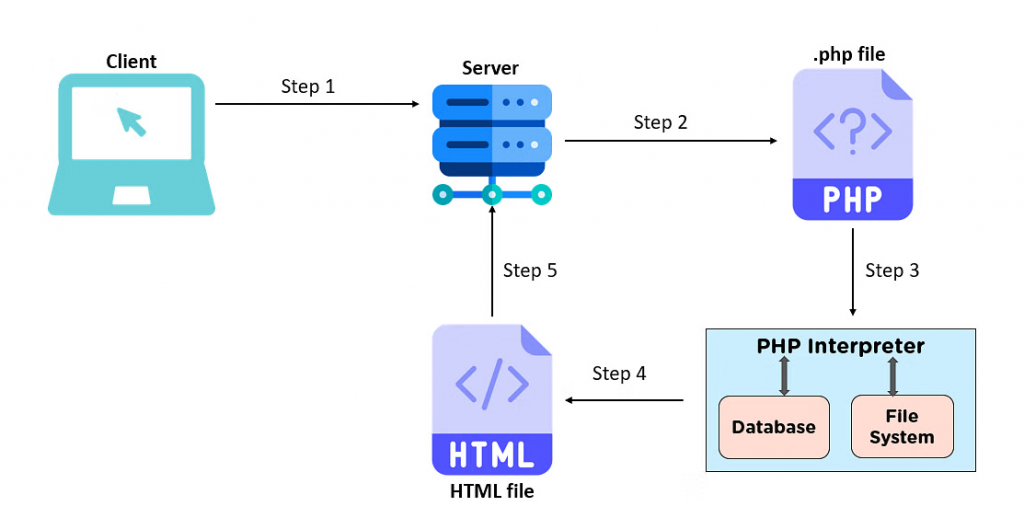
- File Handling
- Reading from and writing to files.
- File manipulation functions.
- File upload handling.
- Database Interaction
- Introduction to databases (e.g., MySQL, PostgreSQL).
- Connecting to a database.
- Executing SQL queries (CRUD operations).
Step 7: Object-Oriented PHP
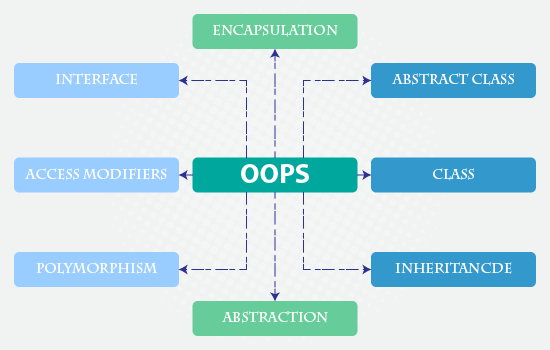
- Classes and Objects
- Defining classes and objects.
- Constructors and destructors.
- Class inheritance and visibility.
- Working with MySQLi or PDO
- Introduction to MySQLi or PDO for database operations.
- Executing prepared statements.
- Error handling and transactions.
Step 8: Web Development Basics
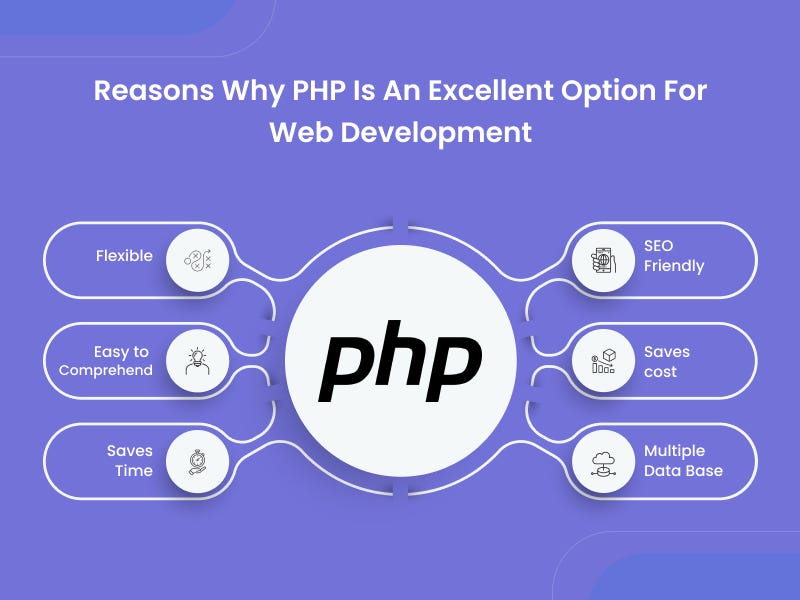
- Introduction to HTML/CSS
- Basics of HTML and CSS.
- Integrating PHP with HTML.
- Sessions and Cookies
- Working with sessions.
- Using cookies for user authentication.
Step 9: Advanced Topics
- Error Handling and Debugging
- Understanding PHP errors and warnings.
- Error logging.
- Debugging techniques.
- Security
- SQL injection prevention.
- Cross-Site Scripting (XSS) prevention.
- Data validation and sanitization.
- Working with APIs
- Consuming external APIs.
- Creating your own APIs.
Step 10: Deployment and Best Practices
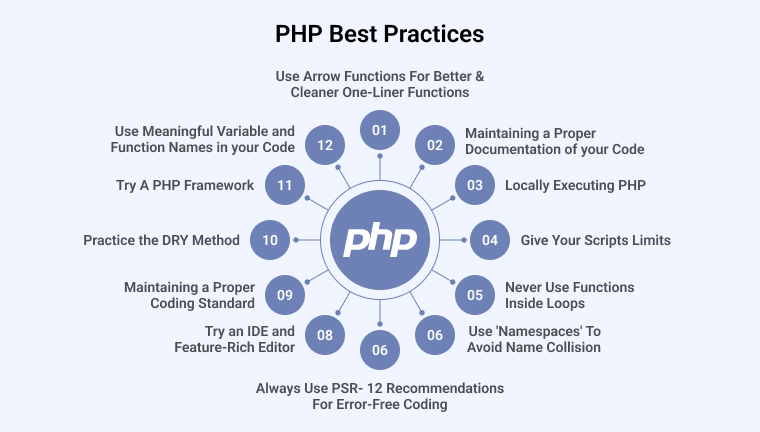
- Deployment
- Uploading your PHP application to a web server.
- Configuration and security considerations.
- Best Practices
- Code organization and readability.
- Performance optimization.
- Version control (e.g., Git).
Step 11: Advanced PHP Frameworks (Optional)
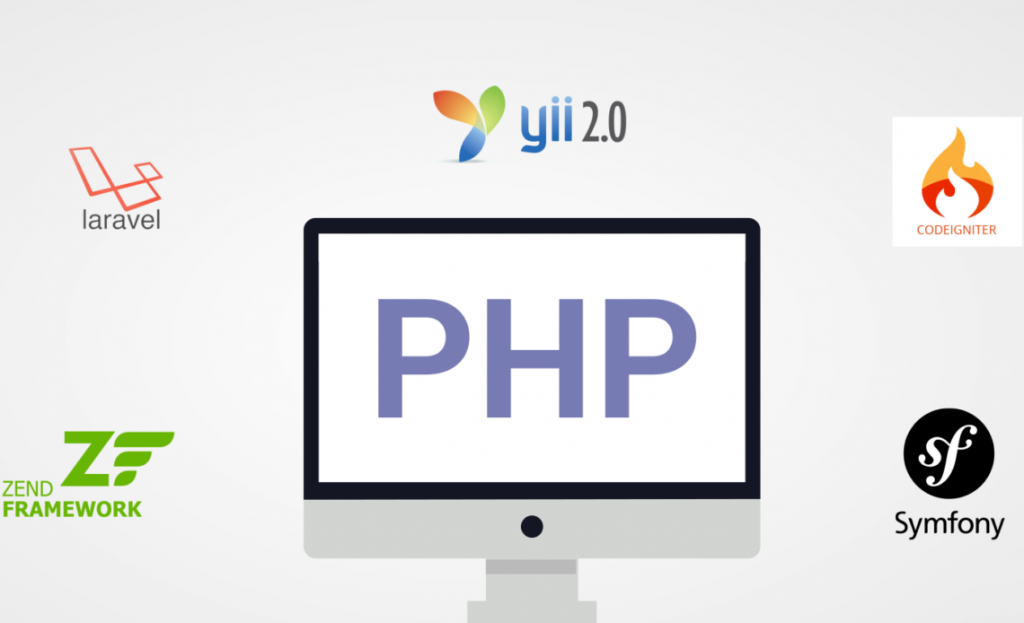
- Introduction to PHP Frameworks
- Overview of popular PHP frameworks (e.g., Laravel, Symfony, CodeIgniter).
- Advantages of using frameworks.
- Building a Project with a Framework
- Setting up a project.
- Routing and MVC architecture.
- Using ORM (Object-Relational Mapping) for database interaction.
Step 12: Continuous Learning
- Resources for Further Learning
- Online tutorials, blogs, and forums.
- Books and documentation.
- Participating in PHP communities and forums.
We build the groundwork for your PHP adventure in Section 1. First, you’ll learn the fundamentals of PHP, including installation and environment setup. To optimize your productivity, we’ll walk you through setting up a local server, installing PHP, and choosing the best text editor or IDE.
The fundamental ideas of PHP are explored in Section 2, which includes syntax, variables, data types, operators, and control structures. Once you have a firm understanding of PHP basics, you will be able to develop clear and effective code.
Functions and arrays, two fundamental PHP building components, are introduced to you in Section 3. You will gain knowledge on how to efficiently deal with arguments and return values, declare and call functions, and work with arrays.
We move our attention to managing form data and file operations in Section 4. You will learn methods for handling form data, confirming user input, and protecting your apps from attacks.
The importance of databases in web development is examined in Section 5. You’ll become an expert at managing database transactions, running SQL queries, and establishing connections to databases.
You will learn about object-oriented PHP in Section 6, which is an effective paradigm for code organization and structure. Discover how to use inheritance and polymorphism for modular and maintainable programs, as well as how to construct classes and generate objects.
We close the gap between front-end technology and PHP in Section 7. Examine the ways in which PHP may be used with HTML, CSS, and JavaScript to create dynamic and engaging websites.
In Section 8, we finally get into advanced subjects and industry best practices. You’ll learn techniques for debugging, safeguarding, and optimizing your PHP apps for maximum efficiency and scalability, from error handling and security to performance optimization.